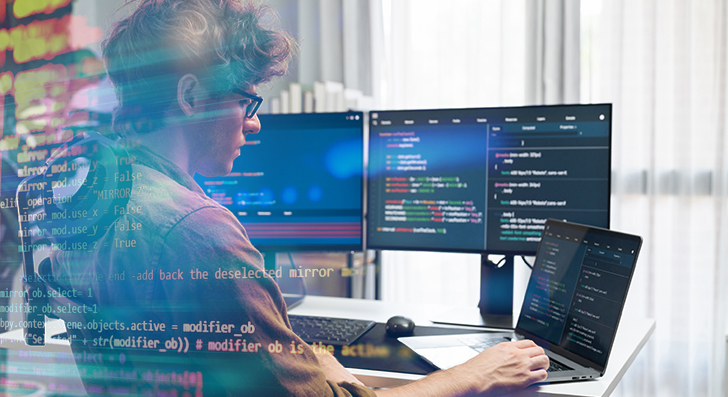
Scalability usually means your software can cope with expansion—a lot more customers, extra facts, and a lot more site visitors—with out breaking. Being a developer, developing with scalability in your mind saves time and stress later on. Right here’s a clear and realistic guidebook that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it should be part of the program from the start. Several purposes fall short every time they expand speedy since the first design and style can’t deal with the additional load. As a developer, you must Imagine early about how your process will behave under pressure.
Commence by building your architecture to get adaptable. Stay away from monolithic codebases wherever every thing is tightly linked. In its place, use modular design and style or microservices. These designs crack your app into lesser, independent elements. Just about every module or service can scale on its own with no influencing The complete method.
Also, think of your database from day a single. Will it will need to take care of one million customers or perhaps a hundred? Select the suitable kind—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
One more significant place is to prevent hardcoding assumptions. Don’t compose code that only operates beneath recent problems. Contemplate what would materialize if your person foundation doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure styles that guidance scaling, like concept queues or function-driven techniques. These aid your application take care of a lot more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you are not just planning for achievement—you are minimizing potential head aches. A well-prepared process is simpler to maintain, adapt, and mature. It’s superior to get ready early than to rebuild afterwards.
Use the best Database
Deciding on the suitable database is a key Component of constructing scalable programs. Not all databases are built exactly the same, and utilizing the Mistaken one can gradual you down and even cause failures as your application grows.
Commence by understanding your facts. Could it be extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is a good healthy. They're strong with associations, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle far more visitors and facts.
In case your facts is more versatile—like user exercise logs, item catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, contemplate your browse and compose designs. Are you carrying out many reads with fewer writes? Use caching and read replicas. Do you think you're managing a heavy generate load? Consider databases that will cope with high compose throughput, as well as party-based info storage programs like Apache Kafka (for momentary data streams).
It’s also sensible to Assume in advance. You might not need Superior scaling characteristics now, but picking a databases that supports them suggests you received’t have to have to change later on.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your facts determined by your access styles. And normally monitor databases performance as you expand.
In brief, the correct database depends upon your app’s structure, velocity requires, And exactly how you be expecting it to improve. Choose time to select correctly—it’ll help save a great deal of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, each and every little delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your method. That’s why it’s crucial to Establish successful logic from the start.
Begin by writing cleanse, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most complicated solution if a straightforward one particular functions. Keep the features short, focused, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take too very long to run or works by using a lot of memory.
Next, evaluate your database queries. These normally sluggish matters down a lot more than the code itself. Be sure each question only asks for the info you actually will need. Stay away from Choose *, which fetches anything, and rather decide on specific fields. Use indexes to speed up lookups. And prevent performing a lot of joins, especially across massive tables.
If you recognize a similar knowledge remaining requested over and over, use caching. Retail outlet the results briefly applying tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, more info batch your database operations when you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application more effective.
Remember to examination with massive datasets. Code and queries that get the job done fine with 100 records could crash every time they have to handle 1 million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These measures support your software remain clean and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional people plus more targeted visitors. If everything goes as a result of a person server, it will immediately turn into a bottleneck. That’s where load balancing and caching come in. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking each of the operate, the load balancer routes consumers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge quickly so it may be reused quickly. When people request the same facts once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it with the cache.
There are two popular varieties of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for fast accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching decreases databases load, improves pace, and makes your app additional effective.
Use caching for things which don’t alter generally. And usually be sure your cache is updated when info does transform.
In brief, load balancing and caching are basic but effective applications. With each other, they help your application handle a lot more people, stay quick, and Get well from complications. If you plan to expand, you require both.
Use Cloud and Container Resources
To develop scalable applications, you will need instruments that permit your application grow very easily. That’s the place cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and providers as you may need them. You don’t really need to obtain components or guess upcoming potential. When targeted visitors increases, you can add more resources with just a few clicks or immediately utilizing auto-scaling. When visitors drops, you are able to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You can focus on building your application in lieu of taking care of infrastructure.
Containers are One more essential Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person device. This causes it to be straightforward to move your application amongst environments, out of your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If 1 component within your app crashes, it restarts it automatically.
Containers also enable it to be simple to separate areas of your app into expert services. You'll be able to update or scale pieces independently, that's great for effectiveness and reliability.
Briefly, utilizing cloud and container applications implies you could scale quickly, deploy effortlessly, and Get better rapidly when challenges occur. In order for you your app to increase without limitations, start out utilizing these instruments early. They save time, lessen hazard, and enable you to keep centered on developing, not repairing.
Observe Every thing
If you don’t check your software, you received’t know when things go Improper. Checking can help the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a important Section of making scalable systems.
Begin by tracking simple metrics like CPU utilization, memory, disk space, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this data.
Don’t just keep track of your servers—keep track of your app also. Keep watch over just how long it requires for consumers to load webpages, how often mistakes take place, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or a company goes down, you'll want to get notified straight away. This can help you correct troubles quickly, frequently before buyers even detect.
Monitoring can also be useful after you make improvements. In case you deploy a different characteristic and see a spike in faults or slowdowns, it is possible to roll it back right before it will cause actual damage.
As your application grows, site visitors and data maximize. With no monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal equipment set up, you remain on top of things.
In a nutshell, monitoring will help you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and making sure it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large providers. Even little applications need a robust Basis. By developing diligently, optimizing wisely, and utilizing the right equipment, you could Develop applications that mature easily devoid of breaking under pressure. Start out small, Consider significant, and Develop sensible.